- Published on
Assigning variable in C
- Authors
- Name
- Ridha Majid
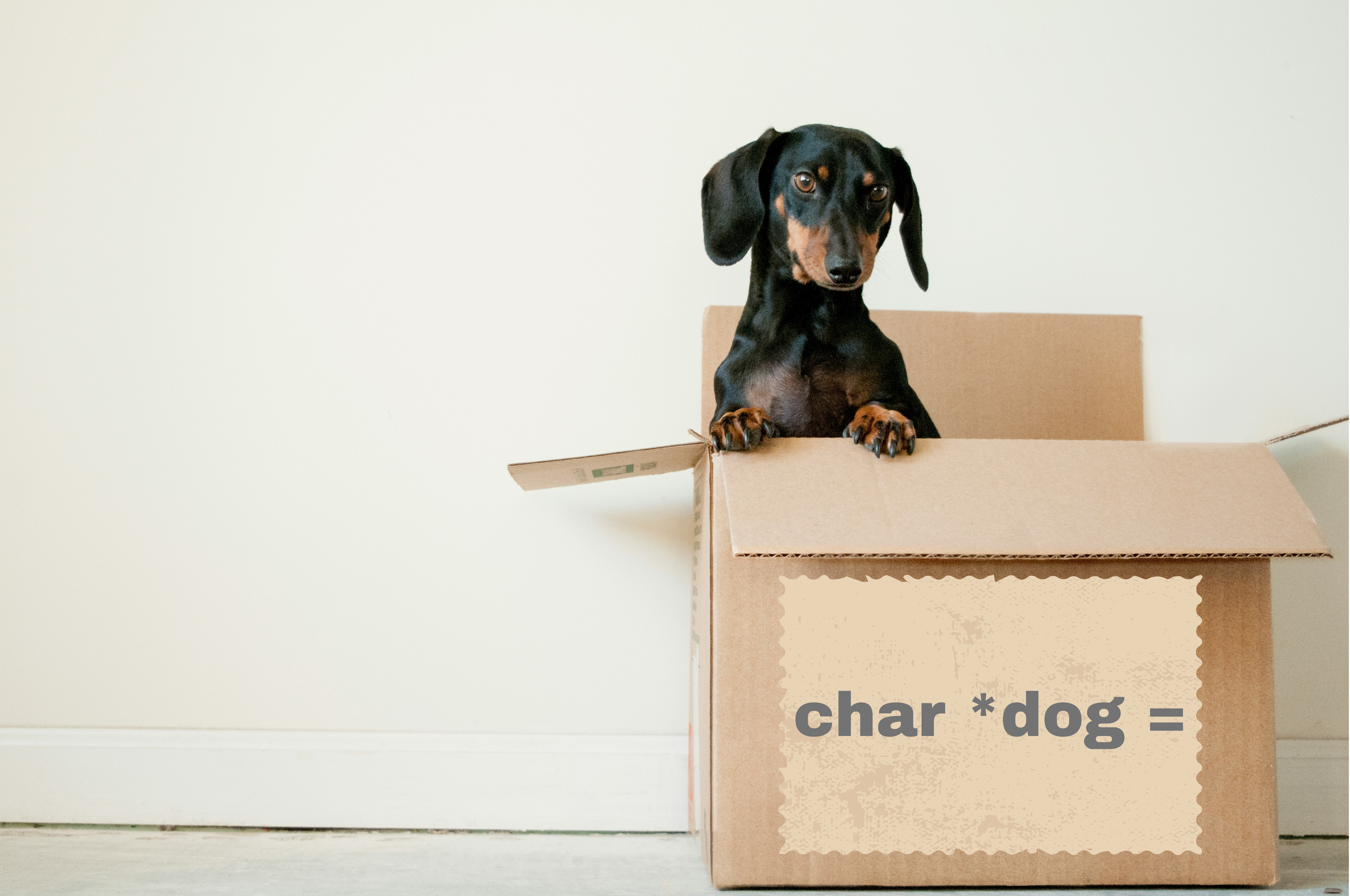
On previous post we've discussed about how make a "hello world" program, now let's we go in deeper how to assigning variable.
According to Wikipedia, Variable is an abstract storage location paired with an associated symbolic name, which contains some known or unknown quantity of data or object referred to as a value.
Integer There are 4 type of variables in C
Integer in C is a data type used to store whole numbers (without decimal points). Integers can be positive, negative, or zero.
We use int
to assigning for the Integer number.
#include <stdio.h>
int main(){
// must put ";"
int hundred = 100;
// `"%i"` is a format specifier used in functions like `printf` in C to print an integer value. The `i` stands for "integer"
printf("%i", hundred);
// output: 100
}
Float
Float data type is use for assigning real number, positive or negative whole number with a decimal point. For example 7.234, -15.678, 0.9321.
We use float
to assigning for the Float number.
#include <stdio.h>
int main(){
// must put ";"
float f = 7.234;
// `"%f"` is a format specifier used in functions like `printf` in C to print an float value. The `f` stands for "float"
printf("%f", f);
// output: 7.234
}
Char
Char data type is use for assigning letter or ASCII Values. you can learn more ASCII Values here.
We use char
to assigning for a char value.
#include <stdio.h>
int main()
{
// must put ";"
// must use single quote ''
char A = 'A';
// 97 is "a" ASCII code.
char a = 97;
// `"%c"` is a format specifier used in functions like `printf` in C to print an float value. The `c` stands for "char"
printf("%c \n", A);
//output: A
printf("%c \n", a);
//output: a
}
String
We're gonna discuss about string more deeper on the next article. For now just keep it mind how to assigned it.
So we use char *variable_name
to assigning for the string value.
The article has been updated. Now you can read the relationship Between Strings and Arrays in here.
#include <stdio.h>
int main(){
// must put ";"
char *hello_world = "Hello world";
// `"%s"` is a format specifier used in functions like `printf` in C to print an string value. The `s` stands for "string"
printf("%s", hello_world);
//output: Hello world
}
BONUS: Use
const
to declare variables whose values cannot be changeduse const
for making the variable unchangeable.
#include <stdio.h>
int main(){
int random_number = 333;
// try to modify a variable
random_number = 44;
// we use const
const int hundred = 100;
// try to modify a variable
hundred = 200;
// `"%i"` is a format specifier used in functions like `printf` in C to print an integer value. The `i` stands for "integer"
printf("%s \n", random_number);
//output: 444
printf("%s \n", hundred);
//output: variable 'hundred' declared const here
}