- Published on
C Arrays. Storing Multiple Values Under One Variable.
- Authors
- Name
- Ridha Majid
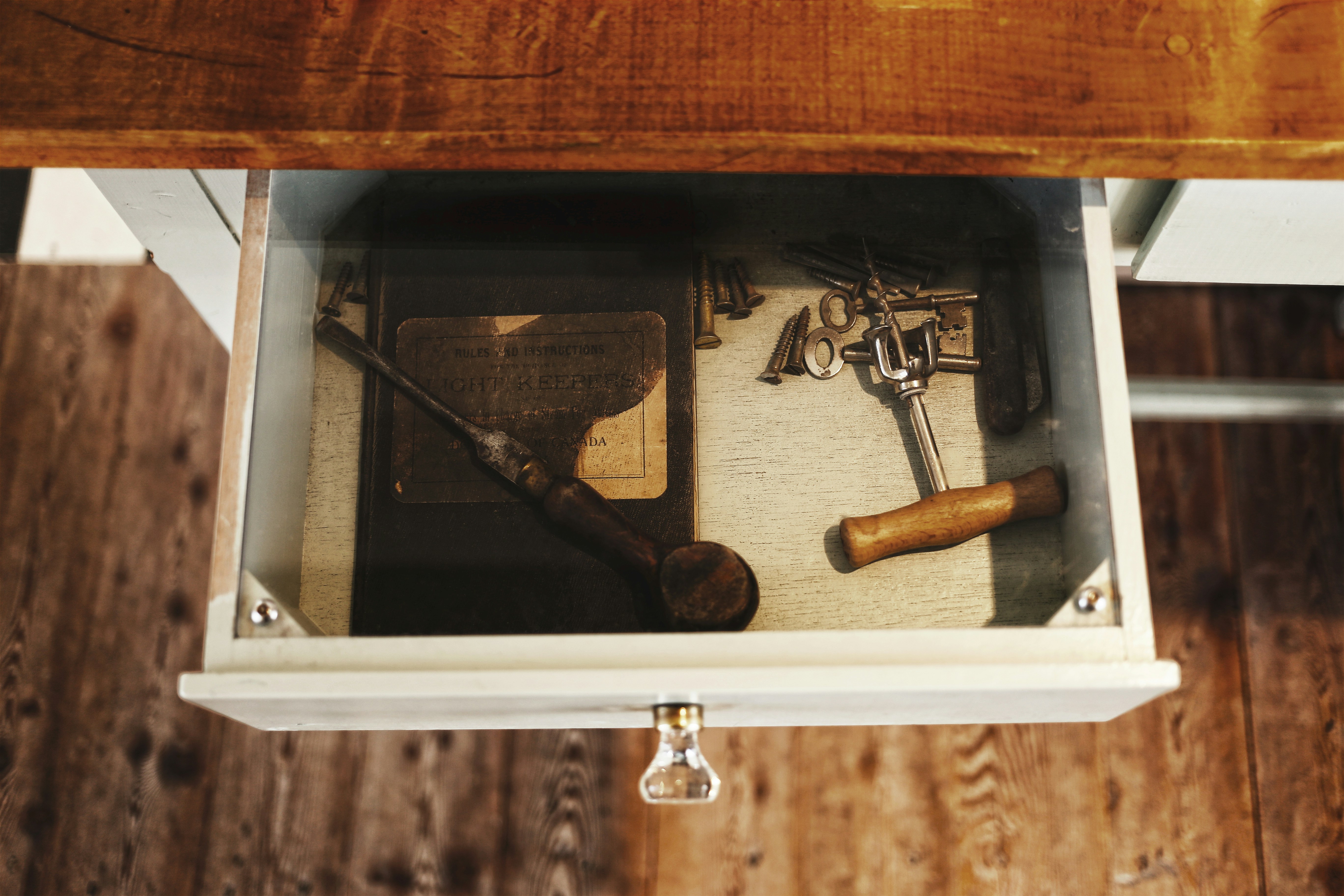
Array is a data type that holds more than one value. It allows you to store multiple values under a single variable name.
In C, arrays are structured like this:
// array_format
type_data array_name[array_size];
Implementing an Array
We are going to implement an array by creating a Date of Birth (DOB) app.
Here's how we do it:
#include <stdio.h>
int date[3];
int main(void)
{
// Input and validate day
do {
printf("Enter day (1-31): ");
scanf("%d", &date[0]);
if (date[0] < 1 || date[0] > 31) {
printf("Invalid day! /n");
}
} while (date[0] < 1 || date[0] > 31);
// Input and validate month
do {
printf("Enter month (1-12): ");
scanf("%d", &date[1]);
if (date[1] < 1 || date[1] > 12) {
printf("Invalid month! \n");
}
} while (date[1] < 1 || date[1] > 12);
// Input and validate year (4 digits)
do {
printf("Enter year (1000-9999): ");
scanf("%d", &date[2]);
if (date[2] < 1000 || date[2] > 9999) {
printf("Invalid year! Please enter a 4-digit year (between 1000 and 9999).\n");
}
} while (date[2] < 1000 || date[2] > 9999);
// Print the validated date of birth
printf("Your date of birth is: %02d/%02d/%d\n", date[0], date[1], date[2]);
}
Relationship Between Strings and Arrays
Let's initialize a string:
char *greeting = "hello-world";
The actual underlying representation looks like this:
['h', 'e', 'l', 'l', 'o', '-', 'w', 'o', 'r', 'l', 'd', '\n']
So, in C, a string is essentially an array of characters.
Let's take a look at a complete example where we can access each character in the string by its index:
#include <stdio.h>
int main(void)
{
char *greeting = "hello-world";
// The actual output would be: ['h', 'e', 'l', 'l', 'o', '-', 'w', 'o', 'r', 'l', 'd', '\n'];
printf("%c\n", greeting[1]); // Output: 'e'
printf("%c\n", greeting[2]); // Output: 'l'
printf("%c\n", greeting[3]); // Output: 'l'
}
In this example, the program prints the characters at the 1st, 2nd, and 3rd positions of the string "hello-world."